Comprehensive Guide to CI/CD with Playwright: Building a Robust Testing Pipeline
The rise of agile methodologies and rapid development cycles has made Continuous Integration and Continuous Deployment (CI/CD) a crucial part of modern software development. CI/CD pipelines automate processes, allowing teams to continuously deliver high-quality software to production environments. When combined with testing frameworks like Playwright, these pipelines ensure that web applications are thoroughly tested before deployment.
In this blog, we’ll take a deep dive into CI/CD pipelines, the role of Playwright in these workflows, and how you can build a robust CI/CD pipeline to automate end-to-end testing of your web applications.
Understanding CI/CD
Let’s break down the core concepts of CI/CD to better understand their importance in the development process:
Continuous Integration (CI)
Continuous Integration is the practice of frequently integrating code into a shared repository. Each time a developer pushes code, automated builds and tests are triggered to ensure that the new code doesn’t break any existing functionality. It allows teams to detect bugs early and prevents integration issues in large projects.
Continuous Deployment (CD)
Continuous Deployment goes a step beyond Continuous Integration. Once the tests are successfully completed, CD automates the release of code to production environments. This ensures that the application is always in a deployable state, enabling rapid and frequent updates with minimal manual intervention.
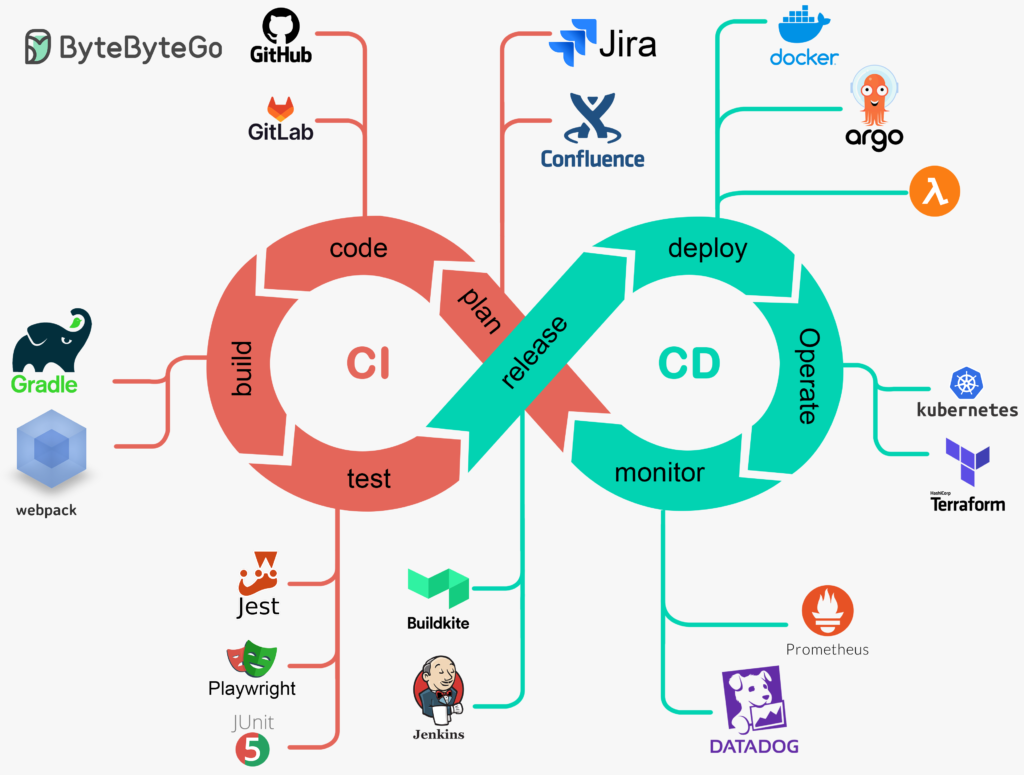
What is Playwright?
Playwright is an open-source automation framework for end-to-end testing of web applications across multiple browsers, including Chromium, Firefox, and WebKit. Built by Microsoft, Playwright is designed to simulate real user interactions with a web page, making it perfect for integration into CI/CD pipelines.
Key Features of Playwright:
Cross-Browser Testing
Playwright supports testing on Chromium, Firefox, and WebKit (Safari).
Parallel Execution
Playwright allows you to run tests in parallel across multiple browser instances, speeding up your test suite.
Headless Mode
Tests can run in headless mode (without a visible browser UI), making them faster and more efficient in CI/CD environments.
Network Interception
Playwright can simulate network conditions and test how your app behaves under different scenarios.
Screenshots and Videos
Capture screenshots and record video clips during test runs to debug failures easily.
Playwright can automate user interactions such as clicks, form submissions, and navigation, making it suitable for regression testing, user acceptance testing, and browser compatibility testing
Why Playwright is Perfect for CI/CD Pipelines
CI/CD pipelines are all about automating the building, testing, and deployment of software. Playwright fits perfectly into these workflows by automating end-to-end testing, ensuring that each code change is tested across multiple browsers before deployment.
Here’s why Playwright is an excellent choice for CI/CD pipelines:
Automated Cross-Browser Testing
Playwright allows you to test your web application across multiple browsers (Chromium, Firefox, WebKit) with minimal configuration.
Headless Testing for Faster Execution
Playwright’s headless mode speeds up test execution, which is essential for CI environments where time is critical.
Parallel Testing
By running tests in parallel, Playwright ensures that large test suites complete in a shorter time
Error Reporting
Playwright provides detailed reports with screenshots and logs, making it easy to debug issues encountered during testing.
Example CI/CD Pipeline Workflow with Playwright
Code Commit
Developers commit code to a shared repository.
Continuous Integration
The CI server (e.g., Jenkins, GitHub Actions) automatically runs a build and triggers Playwright tests.
Automated Testing
Playwright executes tests across different browsers to check the functionality of the web application.
Continuous Deployment
If all tests pass, the code is automatically deployed to the production environment.
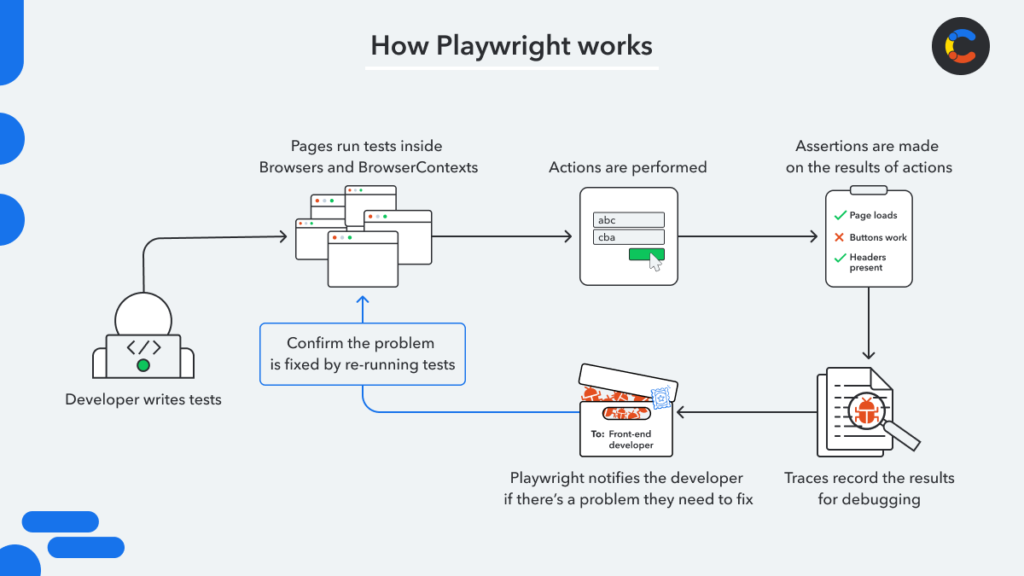
Setting Up a CI/CD Pipeline with Playwright
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
Step 1: Install Playwright
To get started with Playwright, you need to install it in your project. In your terminal, run the following command:
npm install @playwright/test
You can then create your test scripts. Below is an example of a simple Playwright test:
const { test, expect } = require('@playwright/test');
test('homepage has Playwright in title', async ({ page }) => {
await page.goto('https://example.com');
await expect(page).toHaveTitle(/Playwright/);});
Step 2: Configure GitHub Actions for CI/CD
Create a ``.github/workflows/playwright.yml` file in your repository. This file will define your CI/CD pipeline using GitHub Actions.
Here’s an example workflow configuration:
name: Playwright Tests
on:
push:
branches:
- main
pull_request:
branches:
- main
jobs:
test:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Node.js
uses: actions/setup-node@v2
with:
node-version: '16'
- name: Install dependencies
run: npm install
- name: Run Playwright tests
run: npx playwright test
This configuration file triggers Playwright tests on each push or pull request to the `main` branch.
The steps include:
Checking out the code from the repository.
Setting up Node.js as Playwright runs in a Node.js environment.
Installing dependencies required by your project.
Running Playwright tests to ensure the application functions as expected.
Step 3: View Test Results in GitHub Actions
Once you commit and push code to your repository, GitHub Actions will automatically run the tests as per your workflow configuration. You can view the test results in the Actions tab of your repository, where you’ll find detailed logs of each test run, including any errors encountered.
Parallelizing Playwright Tests in CI/CD
Running tests in parallel is one of the most powerful features of Playwright. It allows you to split your test suite across multiple worker threads, drastically reducing the total test execution time.
You can configure Playwright to run tests in parallel by specifying the`workers`option in the Playwright configuration file playwright.config.ts
module.exports = {
use: {
browserName: 'chromium',
},
workers: 4, // Set the number of workers };
In this example, Playwrite will use 4 worker threads to run tests in parallel. This is especially useful in CI environments where time is of the essence.
Best Practices for CI/CD with Playwright
To make the most of Playwright in your CI/CD pipeline, follow these best practices:
Run Tests in Headless Mode: Use headless mode for faster test execution in CI environments. You can enable headless mode in Playwright with the following configuration:
const browser = await playwright.chromium.launch({ headless: true });
Monitor Test Results: Use built-in or third-party tools to generate detailed test reports. Playwright offers an option to generate HTML reports for better visualization of test results.
Parallelize Tests: Take advantage of Playwright’s parallel execution capabilities to reduce test time.
Cross-Browser Testing: Ensure that your Playwright tests cover all major browsers (Chromium, Firefox, and WebKit) to detect browser-specific issues early.
Use Environment Variables: Store sensitive data like API keys in environment variables rather than hardcoding them in your tests. GitHub Secrets is a great way to manage these securely in CI/CD workflows.
Conclusion
Incorporating Playwright into your CI/CD pipeline ensures that your web application is rigorously tested across multiple browsers, reducing the risk of bugs in production. With GitHub Actions (or any other CI/CD tool), you can automate the entire process—from code integration to testing and deployment—while maintaining the quality and reliability of your application.
Playwright’s rich features like parallel testing, cross-browser support, and headless execution make it an ideal choice for CI/CD pipelines, especially in agile development environments where speed and efficiency are paramount.
Start integrating Playwright into your CI/CD pipelines today to boost your team’s productivity, enhance test coverage, and improve software quality.
FAQ,s
- What is Playwright?
Playwright is an open-source framework by Microsoft designed for end-to-end web application testing. It supports cross-browser testing (Chromium, Firefox, WebKit) and multiple programming languages (JavaScript, TypeScript, Python, C#, Java), making it flexible and powerful for automated UI testing. - Which programming languages does Playwright support?
Playwright supports JavaScript/TypeScript, Python, C#, and Java. This multi-language support allows developers to use the language they’re most comfortable with for writing tests. - What browsers does Playwright support?
Playwright supports Chromium (for Chrome and Edge), Firefox, and WebKit (for Safari). This allows for cross-browser compatibility testing on most major platforms. - Is Playwright free to use?
Yes, Playwright is an open-source project under the Apache 2.0 License, making it free to use and modify. - Can Playwright be used for mobile testing?
Yes, Playwright supports mobile emulation, allowing you to simulate different mobile screen sizes and behaviors, but it does not support real-device mobile testing. For that, integration with other mobile testing platforms is typically required. Does Playwright support parallel test execution?
Yes, Playwright supports parallel test execution, which allows tests to run concurrently in multiple browser contexts. This improves test execution speed and is ideal for CI/CD environments.- Can I run Playwright tests in headless mode?
Yes, Playwright can run tests in both headless and headed (with GUI) modes. Headless mode is faster and is typically used in CI/CD pipelines, while headed mode is useful during test development and debugging. How can I handle authentication in Playwright tests?
Playwright allows authentication handling by capturing and reusing authentication cookies or tokens. You can store the authenticated state and reuse it across multiple test sessions, which is efficient for tests requiring user login.Can Playwright be integrated into CI/CD pipelines?
Yes, Playwright integrates well with CI/CD tools like Jenkins, GitHub Actions, GitLab CI, and Azure DevOps. It supports headless execution, parallel testing, and detailed reporting, making it ideal for automated pipelines.Can I use Playwright with existing testing frameworks like Jest or Mocha?
Yes, Playwright can be used with popular testing frameworks like Jest, Mocha, and Pytest. You can install Playwright and configure it to work within these frameworks to take advantage of their testing structures alongside Playwright’s features.